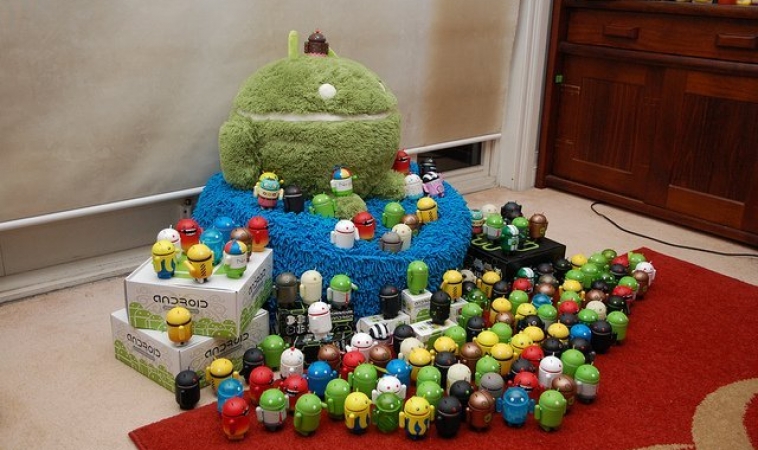
Contents
How To Build Multiple Variants of APKs in Android Studio
Tung Dao Xuan, tungdx1210@gmail.com, is the author of this article and he contributes to RobustTechHouse Blog
Introduction
Have you ever built multiple variants of apk files from a single code base to meet some requirements in programming process, such as: free and paid versions or local backend and remote backend or something like that?
I’ll show you how to do that in Android Studio. In this post, I am going to configure the build process to build one paid version and another free version from a single code base. Both versions have the main screen, but in the free version when users open the program, they will see ads screen before main screen.
Source code for this article can be found at https://github.com/tungdx/MultipleApkEditions.git
Code Structure Of Project
Strings.xml
<resources> <string name="app_name">MultipleApkEditions</string> </resources>
Activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:text="@string/message" android:textSize="20sp" /> </RelativeLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="vn.tungdx.multipleapkeditions"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme"> <activity android:name=".MainActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
MainActivity.java
public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } }
How to configure Android Studio
Step 1: Define product flavors
To define two product flavor, edit the build file for the module to add the following configuration.
productFlavors{ paid{ applicationId "vn.tungdx.multipleapkeditions.paid" } free{ applicationId "vn.tungdx.multipleapkeditions.free" } }
After modifying your build file, sync your Android Studio with the new changes. (Click the below icon)
Open the “Build Variants” view, you will see the result looks like below figure.
Step 2: Create additional directories for each flavor
Paid Program
- On project panel, right-click the src directory under app and select New > Directory.
- Enter “paid” as the name of new directory and click OK
- Create “res” and “values” directories: paid/res/values
- Create xml file, enter the following values:
<resources> <string name="app_name">MultipleApkEditions-Paid</string> <string name="message">In paid version!</string> </resources>
The strings.xml file in “paid” folder will be merged with strings.xml file in code base (main folder)
Free Program
Similar to the paid program, create the following files and directories:
free/res/values/strings.xml
free/res/layout/activity_ads.xml
free/AndroidManifest.xml
free/java/vn/tungdx/multipleapkeditions/AdsActivity.java
strings.xml
<resources> <string name="app_name">MultipleApkEditions-Free</string> <string name="message">Free version!</string> <string name="ads_screen">This is ads screen!</string> <string name="go_to_app">Go to app!</string> </resources>
activity_ads.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:text="@string/ads_screen" /> <Button android:id="@+id/next" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="20dp" android:text="@string/go_to_app" /> </LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="vn.tungdx.multipleapkeditions"> <application> <activity android:name=".AdsActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
This file will be merged with AndroidManifest.xml file in code base (main folder), so in free program version, the ads screen (AdsActivity.java) is run before the main screen (MainActivity.java).
AdsActivity.java
public class AdsActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_ads); findViewById(R.id.next).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent = new Intent(AdsActivity.this, MainActivity.class); startActivity(intent); } }); } }
The resulting directory structure looks like below figure
Step 3: Build project
To work on files from a particular flavor, click on Build Variants on the bottom left of the IDE window and select the variant you want to modify in the Build Variants panel. Android Studio may show errors in source files from flavors other than the one selected in the Build Variants panel, but this does not affect the outcome of the build.
After build project for each flavor, you will get the result as shown below.
Conclusion
That’s it. Hope you can conveniently make multiple variants of apk files that meet your requirements in a short period of time. You can find more info at:
https://developer.android.com/tools/building/configuring-gradle.html
Thanks for reading!
RobustTechHouse is a leading tech company for mobile app development and ECommerce web design and development. If you are interested to engage RobustTechHouse for your projects, you can contact us here.