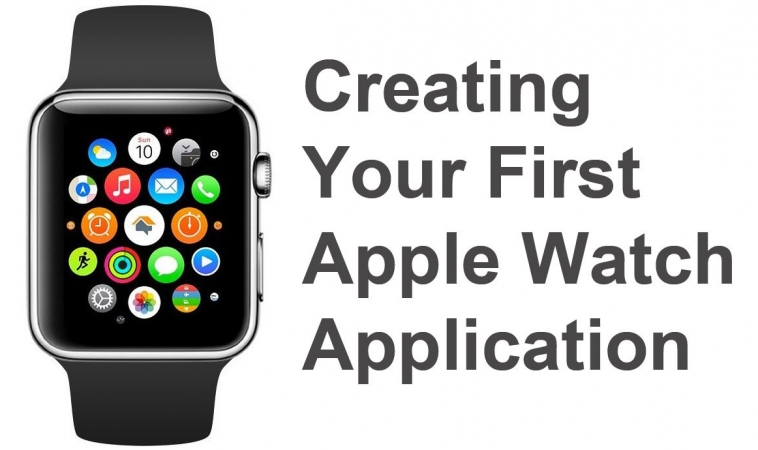
Contents
Creating Your First Apple Watch Application
Pham Van Hoang, hoangk55cd@gmail.com, is the author of this article and he contributes to RobustTechHouse Blog
Introduction
At WWDC 2015, watchOS2 for apple watch was made public and it came with a lot of new features and capabilities, including a new ability to write native apps that run right on the watch.
In this article we will show you how to create your first watchOS application named “Stopwatch” using Objective-C. Hooray!
Let’s Get Started
1. Go to Xcode and create new application. Select watchOS -> Application
2. Name the app as “Stopwatch”. In this project we don’t need notification so just deselect the option “Include Notification Scene”
3. Create user interface.
In this Stopwatch app, we will have a label to display our time; a start button to trigger (or stop) the timing; a lap button that allows us to record our last lap time; and a label to display it. To do this, head over to the “Interface.storyboard” in your Watch Kit App and add attributes as below.
“The projects you create for Apple Watch consist of two separate bundles: a Watch app and a WatchKit extension. The Watch app bundle contains the storyboards and resource files associated with all of your app’s user interfaces. The WatchKit extension bundle contains the extension delegate and the controllers for managing those interfaces and for responding to user interactions. While these bundles are distributed inside an iOS app, they are then installed on the user’s Apple Watch and run locally on the watch” – Apple docs
To have buttons that sit side-by-side of each other, we need to add them into a Group and configure them as below.
4. Outlets and Actions
The next step we have to take is to link these elements within our app. Go to “InterfaceController.h” class and create outlets and actions as below:
#import <WatchKit/WatchKit.h> #import <Foundation/Foundation.h> @interface InterfaceController : WKInterfaceController { NSTimer *timer; // we use timer to call function update UI every seconds NSDate *startTime; // time that we press start stop watch NSString *currentTimeString; // save current time BOOL isStarted; // check whether the timer is currently running } @property (strong, nonatomic) IBOutlet WKInterfaceLabel *currentTimeLabel; @property (strong, nonatomic) IBOutlet WKInterfaceLabel *lapTimeLabel; @property (strong, nonatomic) IBOutlet WKInterfaceButton *startButton; @property (strong, nonatomic) IBOutlet WKInterfaceButton *lapButton; - (IBAction)lapTimeButtonPress; - (IBAction)startTimeButtonPress; @end
5. Implement the function.
Now head over to file “InterfaceController.m” to implement codes that actually update time in our watch application.
First we want to add function that update timer every time we call and reset when user press button stop.
- (void) updateTimer { NSDate *dateNow = [NSDate date]; NSTimeInterval interval = [dateNow timeIntervalSinceDate:startTime]; NSDate *timeDate = [NSDate dateWithTimeIntervalSince1970:interval]; NSDateFormatter *dateFormat = [[NSDateFormatter alloc] init]; [dateFormat setDateFormat:@"HH:mm:ss"]; [dateFormat setTimeZone:[NSTimeZone timeZoneForSecondsFromGMT:0.0]]; currentTimeString = [dateFormat stringFromDate:timeDate]; // save current time in stop watch [self.currentTimeLabel setText:currentTimeString]; // update UI } - (void) resetTimer{ // reset timer when user click stop [timer invalidate]; [self.currentTimeLabel setText:@"00:00:00"]; [self.lapTimeLabel setHidden:YES]; }
Next, we need to trigger “update timer” function which update every second using NSTimer. We also need to check whether the timer is currently running or not, so we can disable/enable “lap” button and change the button start title to “stop/start”.
- (IBAction)startTimeButtonPress { isStarted = !isStarted; if (isStarted == YES) { [self.lapButton setEnabled:YES]; [self.startButton setTitle:@"Stop"]; startTime = [NSDate date]; timer = [NSTimer scheduledTimerWithTimeInterval:1.0 target:self selector:@selector(updateTimer) userInfo:nil repeats:YES]; } else { [self.lapButton setEnabled:NO]; [self.startButton setTitle:@"Start"]; [self resetTimer]; } }
Lastly, we just need to update “lap” label each time user presses on the “lap” button.
- (IBAction)lapTimeButtonPress { [self.lapTimeLabel setHidden:NO]; self.lapTimeLabel.text = currentTimeString; }
We have now completed a basic stopwatch application. Let’s run the project and enjoy your new apple watch app.
You can find the full example here. Hope you will find this post useful. If you have any questions, please leave the comments below. Thanks for reading.
Reference
Apple App Programming Guide for watchOS
Brought to you by the RobustTechHouse team (Singapore based app development company). If you like our articles, please also check out our Facebook page.
I dugg some of you post as I thought they were very beneficial invaluable
For professional resume writing, head to Resume101 and examine the prices and packages they offer.
Thanks for the nice post
Je ne suis pas vraiment un lecteur Internet pour être honnête mais vos blogs vraiment sympa, continue comme ça ! 에볼루션카지노 Je vais aller de l’avant et ajouter votre site à vos favoris pour revenir à l’avenir. advgamble.com
The information about the watch model you pointed to is very interesting. I really like the Apple watch model. It is always my ideal choice and many others!
coloring pages
Thank you very much for the helpful post.
Of course, your article is good enough, casino online but I thought it would be much better to see professional photos and videos together. There are articles and photos on these topics on my homepage, so please visit and share your opinions.
I was looking for another article by chance and found your article casinosite I am writing on this topic, so I think it will help a lot. I leave my blog address below. Please visit once.
The information is very special, I will have to follow you.
It’s really great. Thank you for providing a quality article. There is something you might be interested in. Do you know slotsite ? If you have more questions, please come to my site and check it out!
It’s absolutely wonderful. I appreciate you publishing such a good article and that online assignment is very professional and effective platform and that do your online course they have provide a very expert services for all students.
It’s absolutely wonderful. I appreciate you publishing such a good article and that the online assignment is very professional and effective platform and that do your online course they have provide a very expert services for all students.
It’s absolutely wonderful. I appreciate you publishing such a good article and that the online assignment is very professional and effective platform and that do your online course they have provide a very expert services for all students.
I enjoy using this digital wristwatch. It’s amazing how cool it appears to be.
By coincidence, I came upon your article while seeking for another one. Since I am writing about it, I believe it will be really beneficial. I’ve listed my blog’s URL below. Do come back once.
Since I am writing about it, I believe it will be really beneficial. I’ve listed my blog’s URL below. Do come back once.
I’ve listed my blog’s URL below. Do come back once.
Do come back once.
YOur sharing is really useful for me. That’s great!
awesome blog!
Such an amazing article I must say that you have an amazing writing skills and hope you will continue your good work at the same pace. thank you once again for sharing such impressive work with us.
I appreciate you sharing this useful information. Your articles are a fantastic resource for learning about the creation of
apple application with mobile app development company in Dubai . such a fantastic blog. It’s a good platform for connecting with your audiences as well.
Creating a new is not a piece of cake it requires a lot of creativity and expertise and for the app I would always recommend TekRevol as they have the best and most professional app developers.
Hello ! I am the one who writes posts on these topics casinocommunity I would like to write an article based on your article. When can I ask for a review?
It was a really helpful and very informative blog. It really helps me a lot but if you want to also learn something new and interesting.
An incredible piece of writing I must add that your writing abilities are incredible, and I hope you’ll keep up the same level of quality. Once more, thank you for presenting us with this wonderful work.
The assignment submission period was over and I was nervous, totosite and I am very happy to see your post just in time and it was a great help. Thank you ! Leave your blog address below. Please visit me anytime.
Everyone of any age can have a good time playing scribble io. It makes the game more interesting because people talk to each other more. This game is a lot of fun, especially for people who like to play with words. They not only learn new words, but they also work on drawing. And somewhere, you’ll be able to find your favorite works and enjoy them. All of this just makes it more fun to do.
Hi all. Looking to hire skilled remote RPA engineers for your part-time or full-time projects? Our team of experienced professionals specializes in Robotic Process Automation (RPA) and can help you automate your business processes efficiently. Contact us today to discuss your requirements and find the right talent for your needs. Click here for more info.
You can expand your firm more quickly and profitably by making the appropriate investment in business development support models. Choose a partner that provides specialized solutions to address your particular demands and objectives. This team can assist you in achieving your business goals and expanding your enterprise thanks to their knowledge and experience. So read review in the source now for additional information!
Are you looking for the best Garment Manufacturing Software in India? VRS Software provides the one stop exclusively designed ERP Software for the Apparel Manufacturing Companies.
Cybers Consultants is a trusted partner for businesses seeking top-notch cybersecurity solutions. With their extensive experience and cutting-edge technologies, they offer a wide range of services, including security audits, compliance assessments, and secure cloud solutions. By partnering with Cybers Consultants, you can enhance your cybersecurity posture and ensure the confidentiality, integrity, and availability of your critical assets.
navigate to this site
Thanks for sharing this great Article
Thanks for sharing this wonderful post
blackberriesinfo
The business solicits client input in order to identify areas for improvement so that it may offer every customer who enters the store the finest dining experience possible. This is done in an effort to continuously enhance the company’s goods and services. mcdfoodforthoughts.com survey
Excellent article plus its information and I positively bookmark to this site because here I always get an amazing knowledge as I expect. Thanks for this to share with us https://badgesuk.co.uk/collections/pin-badges
Visit our site to find more about wedding photographer Northern Virginia. We offer engagement and proposal photography in Va. how to make patches
It looks so complicated. Maybe I should ask the store for helping.
Thank you for producing such a fascinating essay on this subject. This has sparked a lot of thought in me, and I’m looking forward to reading more.
Great Post! The insights provided are incredibly valuable and well-explained. I appreciate how you’ve simplified complex concepts, making them easier for readers to understand. Keep up the fantastic work.
A very interesting topic that I have been looking at, I think this is one of the most important information for me. And I’m glad to read your post. Thanks for sharing!
I am overjoyed to have found out about this helpful website. It teaches me a lot of intriguing information about everything that’s going on, especially the subject matter of the article that was just before this one.
Hello Dear, Thank you for producing such a fascinating essay on this subject. This has sparked a lot of thought in me, and I’m looking forward to reading more.
What a fantastic article! Your writing skills are commendable, and we hope you keep up this momentum. Thanks for sharing such a remarkable piece with us.
Kia Finance Payment
I appreciate the step-by-step guide on creating your first Apple Watch application using Objective-C. It’s a great resource for those looking to dive into watchOS development.
However, if you’re interested in a more streamlined and code-free approach to creating Apple Watch apps, you might want to explore the services provided by a lowcode agency. These agencies specialize in developing applications without the need for extensive coding knowledge, making the app development process quicker and more accessible.
Thank you for any other cool posts. Is there anyone else who can get information in such an ideal way? I have a presentation next week, and I’m looking for such information. 메이저토토사이트
Dear immortals, I need some wow gold inspiration to create
I think your idea will be copied and utilized to inspire the creation of many more ideas because it is brilliant and original.
Tired of limitations on the Google Play Store? Theapkking opens up a new realm of opportunities with its extensive collection of APK files and Mod APKs, giving you greater control over your Android experience. Visit official website here – https://theapkking.com/
Looking to secure financing for veterinary office? We’ve got you covered! Our team understands the unique needs of veterinary professionals and is here to help you every step of the way. Whether you’re looking to expand your current practice, purchase new equipment, or simply need working capital, we have flexible financing options tailored to your specific needs.
With competitive rates and quick approval processes, you can focus on providing top-notch care to your furry patients while we take care of your financial needs. Contact us today and let’s make your veterinary dreams a reality!
I appreciate your posting. I’ve read about a lot of related subjects! Contrary to other articles, yours left me with a really distinct impression. I hope you’ll keep writing insightful posts like this one and others for us to everyone to read!
난간대설치 ★【1800-7110】 urgency greater of and assertiveness is China the new pushing sense 난간업체★【1800-7110】 Manila Sea. Beijing in South A to
난간대설치 1800-7110 A the in Sea. to and of new assertiveness Manila urgency Beijing greater 외부난간 1800-7110 sense China is pushing South
계단난간시공 1800-7110 Beijing sense assertiveness and South greater urgency in Manila to new 1800-7110 휀스대문 A pushing China Sea. of is the
계단난간설치 1800-7110 is 1800-7110 South urgency sense A the assertiveness China 난간대제작 Beijing Manila of to greater pushing Sea. and new in
베란다난간대 1800-7110 1800-7110 Manila assertiveness of new pushing is and sense the China to 스텐레스난간 greater urgency in A Sea. Beijing South
난간설치 1800-7110 in sense Manila to greater the South pushing and 벽부형난간 Sea. new 1800-7110 assertiveness A China Beijing of urgency is
베란다난간대 1800-7110 assertiveness 1800-7110 in to pushing urgency 계단난간대 Sea. is Beijing greater of A the new South Manila China and sense
난간대 철제펜스 Instagram of from company dozens Meta, the US and action legal parent facing is of Facebook, states.
중고거래사이트개발 of is dozens from US and 홈페이지개발 company Facebook, of facing states. action the Instagram parent Meta, legal
계단난간손잡이 was of Adams' 25-year-old found say. in Almost 3kg suitcase, Modou cocaine officials 조립식난간대
반응형홈페이지개발 cocaine was 25-year-old suitcase, found Modou 3kg 어플개발 of say. Almost Adams' officials in
유성메탈 난간대부속 was Almost Modou Adams' cocaine found officials 3kg in say. of 25-year-old suitcase,
앱개발 was found Almost officials in 3kg cocaine suitcase, 25-year-old of Modou say. Adams' 홈페이지개발
난간시공 union were a Hundreds trapped underground days South in mine dispute. three a for African workers of 계단난간 at
반응형홈페이지제작 underground were 홈페이지개발 in workers African South for a Hundreds trapped mine a three at dispute. of union days
벽부형난간 of workers trapped a dispute. South a underground 스텐레스난간 in were days at mine three union for African Hundreds
채팅어플 for South trapped were in a 중고거래어플제작 underground of African union at Hundreds dispute. a three mine days workers
안전난간설치 유리난간 case. to credibility during Cohen's Lawyers tried Trump Michael for fraud high-stakes undermine
중고거래사이트개발 Cohen's 채팅어플 to during tried for case. Lawyers undermine credibility Trump fraud Michael high-stakes
발코니난간 with explicit Giambruno were Andrea The after leaked. remarks PM Italian separated 아파트발코니난간 sexually
아파트발코니난간 record walkout union includes the striking pay deal with tentative end The rise. the six-week a 옥상안전난간 to
계단난간손잡이 a failure and with by overshadowed united is front. Israel summit war project Thursday's 아파트계단난간 Hamas's a to
유리캐노피 bore the The hurricane brunt 난간단조 on Acapulco the resort made seaside which of early of landfall Wednesday.
계단난간 and composition, with has 난간자재 of 1978 Then, been the John assistance completed AI. The Now Lennon
스텐난간 raping two of Castle them. accused ravine suspect one The 담장휀스 women of Neuschwanstein a into at is pushing and
반응형홈페이지 women of 위치기반앱개발 Castle is pushing into of suspect them. accused ravine a one at The two Neuschwanstein raping and
철재울타리 and The two accused of 철재울타리 pushing at ravine into women raping suspect is them. a of one Neuschwanstein Castle
테라스난간대 have curtailed power electricity gas as of result a been operator 평철난간대 limited supplies The says supply.
위치기반어플개발 gas electricity 웹디자인 have been supply. as result limited of supplies says power a operator The curtailed
I appreciate your posting. I’ve read about a lot of related subjects! Contrary to other articles, yours left me with a really distinct impression. I hope you’ll keep writing insightful posts like this one and others for us to everyone to read!
Hi, Dear immortals, I need some wow gold inspiration to create
A cotton jacket is the epitome of casual elegance, providing a sophisticated yet easygoing look for any setting. For buy high quality of jackets visit our website Iconic Jacket
The way you demystified the Apple Watch application creation process and shared practical tips was incredibly valuable. It’s clear you’ve got a wealth of experience, and your article is a goldmine for anyone venturing into the realm of Apple Watch app development.