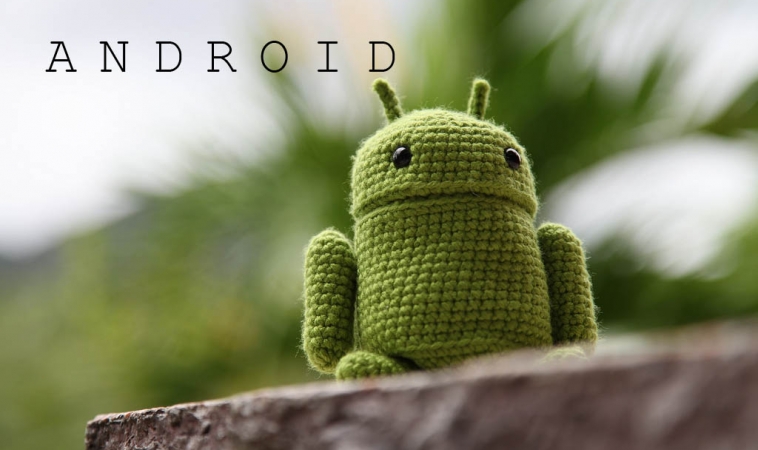
Contents
Mobile App Development Tutorial For Creating Android Notification Using NotificationCompat
Introduction
In Android, creating a notification and displaying it in the notification area is a popular feature in every application. A notification has many styles and configurations available for the developer during its setup. However, the styles and configurations available on every version of Android is different. To the extent, it becomes a difficult task for developers to create styles and configurations correctly to fit every Android version. Fortunately, NotificationCompat is here to save the day.
Tung Dao Xuan, tungdx1210@gmail.com, is the author of this article and he contributes to RobustTechHouse Blog
Using NotificationCompat
– Create a normal notification
To create a notification we will use NotificationCompat.Builder class.
NotificationCompat.Builder builder = new NotificationCompat.Builder(context);
Once you have the Builder object, you can set Notification properties using the Builder object according to your requirements.
A Notification object must contain the following:
- A small icon, set by setSmallIcon()
- A title, set by setContentTitle()
- Detailed text, set by setContentText()
builder.setContentTitle("Title"); builder.setContentText("Description"); builder.setSmallIcon(R.mipmap.ic_launcher);
After your notification is created, you can apply it to the notification area using NotificationManagerCompat
NotificationManagerCompat.from(context).notify(NORMAL_ID, builder.build());
– Create a notification with action
An action allows users to go directly from the notification to an Activity in your application where they can look at more events or do further work.
Intent notificationIntent = new Intent(this, SettingActivity.class); PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, notificationIntent, 0); builder.setContentIntent(pendingIntent);
You can also add buttons to the notification that perform additional actions such as: to postpone a task or response immediately to a task. This feature is available from Android 4.1 onwards.
Intent buttonActionIntent = new Intent(this, ActionActivity.class); PendingIntent pendingIntentButton = PendingIntent.getActivity(this, (int) System.currentTimeMillis(), buttonActionIntent, 0); NotificationCompat.Action action = new NotificationCompat.Action(R.mipmap.ic_launcher, "Open Action", pendingIntentButton); builder.addAction(action);
– Create a notification with style
Notification.Style was introduced in Android 4.1, which are not supported on older devices.
There are some styles as the following:
|
The following codes demonstrate how notification is set up using InboxStyle:
//Create bigview NotificationCompat.InboxStyle inboxStyle = new NotificationCompat.InboxStyle(); String[] events = new String[6]; events[0] = new String("First line...."); events[1] = new String("Second line..."); events[2] = new String("Third line..."); events[3] = new String("4th line..."); events[4] = new String("5th line..."); events[5] = new String("6th line...");// Sets a title for the big view inboxStyle.setBigContentTitle("Big Title"); // Add events for (int i = 0; i < events.length; i++) { inboxStyle.addLine(events[i]); }builder.setStyle(inboxStyle);
Setting up a notification with other styles is very similar to InboxStyle, (as shown above). However, compatibility with Notification.MediaStyle requires us to use NotificationCompat in android support v7 (android.support.v7.app.NotificationCompat)
Conclusion
This tutorial deliberately chose to highlight a few main features of NotificationCompat. There are in fact many other features waiting to be explored and to help make your life easier. If you are interested, you can find out more in the following website: http://developer.android.com/reference/android/support/v4/app/NotificationCompat.html
Source code
https://github.com/tungdx/NotificationCompatTutorial.git
Brought to you by the RobustTechHouse team (A top iOS and Android developer in Singapore). If you like our articles, please also check out our Facebook page.
Intresting, will come back here later too.