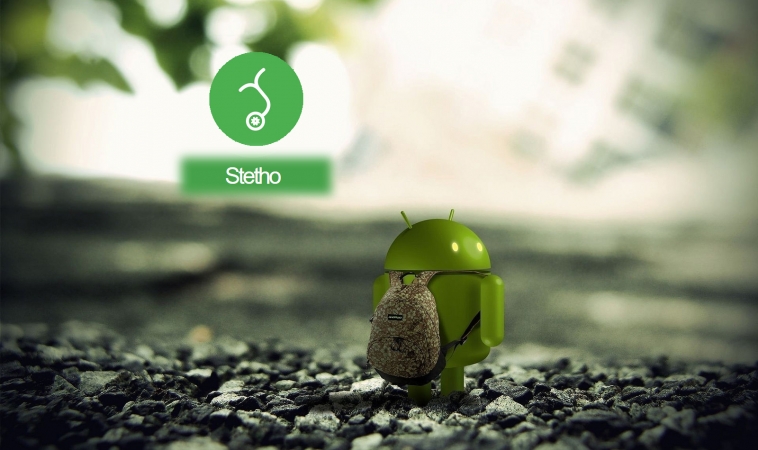
Contents
Debugging Android using Facebook Stetho Library
Tung Dao Xuan, tungdx1210@gmail.com, is the author of this article and he contributes to RobustTechHouse Blog
Introduction
Stetho is a debug bridge for Android applications, enabling the powerful Chrome Developer Tools and much more. Check it from here.
In this tutorial, you are going to learn how to integrate an Android project with Stetho (version 1.3.1) and use Google Chrome’s developer tools to debug it.
How to setup
- Gradle
compile 'com.facebook.stetho:stetho:1.3.1'
- Initializing Stetho
Create a class that extends Application and initialize Stetho inside its onCreate() method.
public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); Stetho.initializeWithDefaults(this); } }
In AndroidManifest.xml file, add MyApplication class into application tag as following:
<application android:name=".MyApplication" </application>
Once you complete the set-up instructions above, just start your app and point your laptop browser to ‘chrome://inspect’. Click the “Inspect” button to begin.
Your browser
- Inspecting databases
Click the Resources tab and select Web SQL. If your app has any SQLite databases, they will be listed here. Selecting a database shows a list of the tables in the database. Finally, clicking a table displays the records of the table:
- Inspecting preferences
Open the Resources tab of the Developer Tools window and select LocalStorage. You will see the names of the files your app uses to store the preferences. You can even edit the values stored in a file.
- Inspecting network connections
If you are using the popular OkHttp library in the 2.2.x+ or 3.x release, you can use the ‘Interceptors system’ to automatically hook into your existing stack. This is currently the simplest and most straightforward way to enable network inspection.
Add dependency
compile 'com.facebook.stetho:stetho-okhttp3:1.3.1'
Add Stetho network interceptor into OkHttpClient as follows:
OkHttpClient client = new OkHttpClient.Builder().addNetworkInterceptor(new StethoInterceptor()).build();
The following is the source code to call the Api.
private void callApi() { OkHttpClient client = new OkHttpClient.Builder().addNetworkInterceptor(new StethoInterceptor()).build(); Request request = new Request.Builder().url("https://api.myjson.com/bins/1gi9v").build( ); client.newCall(request).enqueue(new Callback() { @Override public void onFailure(Call call, IOException e) { Log.i(TAG, "call api error"); } @Override public void onResponse(Call call, Response response) throws IOException { if (!response.isSuccessful()) throw new IOException("Unexpected code " + response); final String body = response.body().string(); runOnUiThread(new Runnable() { @Override public void run() { mResponseView.setText(body); } }); } }); }
Open the Network tab of the Developer Tools window, you will see as following result:
To inspect images loaded, you must add Stetho network interceptor into your used image library. In this tutorial, we use Pisasso library for loading images from a server.
Add dependencies
compile 'com.squareup.picasso:picasso:2.5.2' compile 'com.jakewharton.picasso:picasso2-okhttp3-downloader:1.0.2'
Configure Piscasso library with Stetho
private void initPicasso() { OkHttpClient picassoClient = new OkHttpClient.Builder().addNetworkInterceptor(new StethoInterceptor()).build(); Picasso picasso = new Picasso.Builder(this).downloader(new OkHttp3Downloader(picassoClient)).build(); Picasso.setSingletonInstance(picasso); }
When you load an image from a server, you can see as following
Picasso.with(this).load("http://www.wholesale7.net/images/201310/goods_img/115013_P_1381825983592.jpg").into(mImageView);
Conclusion
With Stetho library for debugging you can shorten your development time. We tried to be as concise in our tutorial but there are some other Stetho features that are not covered in this tutorial, you can find them here.
I hope you found this tutorial useful, and don’t forget to share it if you liked it.
Source code
https://github.com/tungdx/FacebookStethoTutorial
Brought to you by the RobustTechHouse team (A top app development company in Singapore). If you like our articles, please also check out our Facebook page.
Deference to op , some superb selective information .