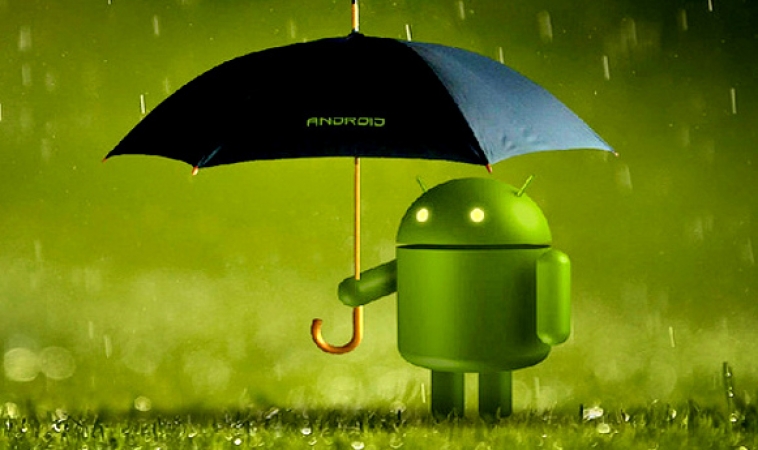
Contents
Android Runtime Permission in Android 6.0 Marshmallow
Tung Dao Xuan, tungdx1210@gmail.com, is the author of this article and he contributes to RobustTechHouse Blog
If the device is running Android 6.0 or higher and your app’s target SDK is 23 or higher, then the mobile app has to list the permissions in its manifest; it must request each dangerous permission to the user it needs while the app is running. The user can grant or deny these permissions and the app can continue to run with limited capabilities even if any of the request is denied. .
This tutorial shall show you how to handle your app development correctly when working with runtime permissions in Android 6.0
Coding
This tutorial creates an app with two screens. When the user clicks a button on the first screen, the app will check if the “READ_CONTACTS” permission is granted or not. If the permission is not granted for this app, it will request to the user for permission before displaying the contact list which is the second screen we were alluding to earlier.
- Check For Permissions
ActivityCompat.checkSelfPermission(this, Manifest.permission.READ_CONTACTS) != PackageManager.PERMISSION_GRANTED
Check if the Camera permission is already available.
If the app has gotten the permission, the method returns ‘PackageManager.PERMISSION_GRANTED’ and the app can proceed with the operation. If the app does not have the permission, the method returns ‘PERMISSION_DENIED’, then the app has to explicitly ask the user for the relevant permission.
- Request Permissions
private void requestContactsPermissions() { // Should we show an explanation? if (ActivityCompat.shouldShowRequestPermissionRationale(this, Manifest.permission.READ_CONTACTS)) { // Show an expanation to the user and try again to request the permission. AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setMessage(R.string.permission_contacts_rationale); builder.setPositiveButton(R.string.ok, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { ActivityCompat .requestPermissions(MainActivity.this, new String[]{Manifest.permission.READ_CONTACTS}, REQUEST_CONTACTS); } }); builder.setNegativeButton(R.string.cancel, null); builder.show(); } else { // No explanation needed, we can request the permission. ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.READ_CONTACTS}, REQUEST_CONTACTS); } }
Android provides a utility method, ‘shouldShowRequestPermissionRationale()’, which returns true if the app has requested this permission previously but was denied by the user. In this case we should show an explanation to let the users know why this app needs this permission.
This app passes the permissions it wants and also an integer request code that the developer specifies to identify this permission request.
- Handle the permissions request response
When this app requests permissions, the system presents a dialog box to the user. When the user responds, the system invokes the app’s ‘onRequestPermissionsResult()’ method, passing back to the app user’s response.
@Override public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) { if (requestCode == REQUEST_CONTACTS) { if (verifyPermissions(grantResults)) { openContactsActivity(); } else { Toast.makeText(MainActivity.this, R.string.contacts_permission_not_granted, Toast.LENGTH_SHORT).show(); } } else { super.onRequestPermissionsResult(requestCode, permissions, grantResults); } }
This app has to override that method to find out whether the permission is granted. The callback is passed the same request code you have passed to ‘requestPermissions()’.
When the permission is granted, the developer can proceed to perform the task that it needs to do.
Conclusion
That’s it! The above are the few steps you need do when working with new model permission in Android 6.0 onward.
We hope that you have found this tutorial useful as much as we enjoy writing it.
Source code
https://github.com/tungdx/AndroidRuntimePermissionsTutorial
Brought to you by the RobustTechHouse team (A top app development company in Singapore). If you like our articles, please also check out our Facebook page.
It’s great that this site has a frequently asked questions section. شركة تركيب طارد الحمام بالرياض